1 - Sign PDF with certificate from file or upload.
<?php
use Illuminate\Http\Request;
use LSNepomuceno\LaravelA1PdfSign\Sign\{ManageCert, SignaturePdf};
class ExampleController() {
public function dummyFunction(Request $request){
try {
$cert = new ManageCert;
$cert->fromPfx('path/to/certificate.pfx', 'password');
} catch (\Throwable $th) {
}
try {
$cert = new ManageCert;
$cert->fromUpload($request->pfxUploadedFile, $request->password);
dd($cert->getCert());
} catch (\Throwable $th) {
}
try {
$pdf = new SignaturePdf('path/to/pdf/file.pdf', $cert, SignaturePdf::MODE_RESOURCE)
$resource = $pdf->signature();
} catch (\Throwable $th) {
}
try {
$pdf = new SignaturePdf($request->file('PDFfile'), $cert, SignaturePdf::MODE_RESOURCE)
$resource = $pdf->signature();
} catch (\Throwable $th) {
}
try {
$pdf = new SignaturePdf('path/to/pdf/file.pdf', $cert, SignaturePdf::MODE_DOWNLOAD)
return $pdf->signature();
} catch (\Throwable $th) {
}
}
}
2 - Sign PDF with certificate from database (model based).
<?php
use Illuminate\Http\Request;
use App\Models\Certificate;
use LSNepomuceno\LaravelA1PdfSign\Sign\{ManageCert, SignaturePdf};
class ExampleController() {
public function dummyFunction(Request $request){
$cert = Certificate::find(1);
try {
$pdf = new SignaturePdf('path/to/pdf/file.pdf', $cert->parse(), SignaturePdf::MODE_RESOURCE)
$resource = $pdf->signature();
} catch (\Throwable $th) {
}
try {
$pdf = new SignaturePdf('path/to/pdf/file.pdf', $cert->parse(), SignaturePdf::MODE_DOWNLOAD)
return $pdf->signature();
} catch (\Throwable $th) {
}
}
}
3 - Sign PDF with image insertion
<?php
use Illuminate\Http\Request;
use App\Models\Certificate;
use Illuminate\Support\Facades\File;
use LSNepomuceno\LaravelA1PdfSign\Sign\{ManageCert, SealImage, SignaturePdf};
class ExampleController() {
public function dummyFunction(Request $request){
try {
$cert = new ManageCert;
$cert->fromPfx('path/to/certificate.pfx', 'password');
} catch (\Throwable $th) {
}
try {
$cert = new ManageCert;
$cert->fromUpload($request->pfxUploadedFile, $request->password);
dd($cert->getCert());
} catch (\Throwable $th) {
}
$image = SealImage::fromCert($cert);
$imagePath = a1TempDir(true, '.png');
File::put($imagePath, $image);
try {
$pdf = new SignaturePdf('path/to/pdf/file.pdf', $cert)
$resource = $pdf->setImage($imagePath)
->signature();
} catch (\Throwable $th) {
}
}
}
3.1 - The "setImage" method
<?php
[...]
public function setImage(
string $imagePath, // Image path location
float $pageX = 155, // X page position
float $pageY = 250, // Y page position
float $imageW = 50, // The image width, if set to 0, the original or proportional image size will be used
float $imageH = 0 // The image height, if set to 0, the original or proportional image size will be used
): SignaturePdf
[...]
3.2 - The expected result will be as shown below
IMPORTANT
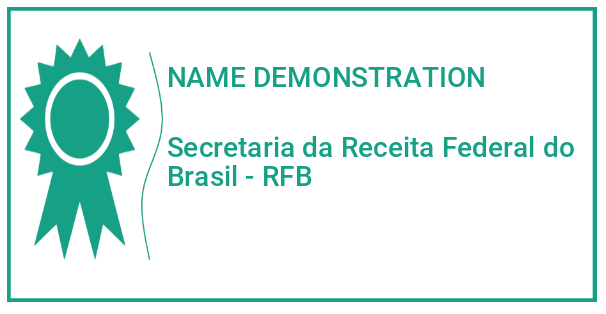
3.3 - In some cases you may need to create your own signature seal, check the implementation of the SealImage::fromCert method for details.
<?php
[...]
try {
$pdf = new SignaturePdf('path/to/pdf/file.pdf', $cert)
$resource = $pdf->setImage($imagePath)
->setFileName('new-file-name.pdf')
->setInfo(
$name,
$location,
$reason,
$contactInfo
)
->setHasSignedSuffix(false)
->signature();
} catch (\Throwable $th) {
}
[...]